PowerShell Get-WMIObject | Get-Ciminstance
What is WMI?
WMI (Windows Management Instrumentation) is a Windows administration feature that provides a standardized interface for querying and interacting with various components of the operating system, hardware, and software.
Using PowerShell with WMI
PowerShell is a powerful scripting language and command-line shell that allows you to interact with WMI easily. You can use cmdlets like Get-WmiObject (in PowerShell 5.1 and earlier) or Get-CimInstance (in PowerShell 3.0 and later) to query WMI classes.
​
WMI Classes
​
1. Win32_BIOS
This class provides information about the BIOS of the computer system.
# Get-CimInstance BIOS Information
$bios = Get-CimInstance -ClassName Win32_BIOS
Write-Output "BIOS Information:"
Write-Output "Manufacturer: $($bios.Manufacturer)"
Write-Output "Name: $($bios.Name)"
Write-Output "Version: $($bios.Version)"
Write-Output "Release Date: $($bios.ReleaseDate)"
Write-Output ""
​
​
# # Get-CimInstance Computer System Information
$computerSystem = Get-CimInstance -ClassName Win32_ComputerSystem
Write-Output "Computer System Information:"
Write-Output "Manufacturer: $($computerSystem.Manufacturer)"
Write-Output "Model: $($computerSystem.Model)"
Write-Output "Total Physical Memory: $($computerSystem.TotalPhysicalMemory)"
Write-Output "User Name: $($computerSystem.UserName)"
Write-Output ""
​
​
# Get-CimInstance Network Adapter Configuration
$networkAdapters = Get-CimInstance -ClassName Win32_NetworkAdapterConfiguration | Where-Object { $_.IPEnabled -eq $true }
Write-Output "Network Adapter Configuration:"
foreach ($adapter in $networkAdapters) {
Write-Output "Description: $($adapter.Description)"
Write-Output "MAC Address: $($adapter.MACAddress)"
Write-Output "IP Address: $($adapter.IPAddress -join ', ')"
Write-Output "Default IP Gateway: $($adapter.DefaultIPGateway -join ', ')"
Write-Output ""
}
​
​
# Get-CimInstance Installed Products
$products = Get-CimInstance -ClassName Win32_Product
Write-Output "Installed Products:"
foreach ($product in $products) {
Write-Output "Name: $($product.Name)"
Write-Output "Version: $($product.Version)"
Write-Output "Vendor: $($product.Vendor)"
Write-Output "Install Date: $($product.InstallDate)"
Write-Output ""
}
# Using Get-WmiObject (for older versions of PowerShell)
Get-WmiObject -Class Win32_BIOS
# Using Get-CimInstance (recommended for newer versions of PowerShell)
Get-CimInstance -ClassName Win32_BIOS
Win32_BIOS Common Properties:
-
Manufacturer: The name of the BIOS manufacturer.
-
Name: The name of the BIOS.
-
Version: The version of the BIOS.
-
ReleaseDate: The release date of the BIOS.
​
​
​
2. Win32_ComputerSystem
This class represents a computer system running on a Windows operating system.
# Using Get-WmiObject
Get-WmiObject -Class Win32_ComputerSystem
# Using Get-CimInstance
Get-CimInstance -ClassName Win32_ComputerSystem
Win32_ComputerSystem Common Properties:
-
Manufacturer: The name of the computer manufacturer.
-
Model: The model of the computer.
-
TotalPhysicalMemory: The total physical memory available in the system.
-
UserName: The name of the user currently logged on.
​
​
3. Win32_NetworkAdapterConfiguration
This class represents the attributes and behaviors of a network adapter.
# Using Get-WmiObject
Get-WmiObject -Class Win32_NetworkAdapterConfiguration
# Using Get-CimInstance
Get-CimInstance -ClassName Win32_NetworkAdapterConfiguration
Common Properties:
-
Description: A description of the network adapter.
-
MACAddress: The MAC address of the network adapter.
-
IPAddress: The IP address(es) assigned to the adapter.
-
DefaultIPGateway: The default IP gateway for the adapter.
​
​
4. Win32_ProductThis class represents products
as they are installed by Windows Installer.
# Using Get-WmiObject
Get-WmiObject -Class Win32_Product
# Using Get-CimInstance
Get-CimInstance -ClassName Win32_Product
Common Properties:
-
Name: The name of the installed product.
-
Version: The version of the installed product.
-
Vendor: The vendor or publisher of the product.
-
InstallDate: The installation date of the product.
Get-WMIObject & Get-Ciminstance Examples:
Combine WMI Data with PSCustom Object PS Ver. 3
# Use WMI get IP on My Subnet
$IPAddress = Get-WmiObject Win32_NetworkAdapterConfiguration | Where{$_.IPAddress -Like "192.168.254*"} | Select -ExpandProperty IPAddress
# Use WMI get Operating System
$OS = (Get-WmiObject Win32_Operatingsystem ).Version
# Get-Host PowerShell Version
$PSVersion= (Get-Host | Select -ExpandProperty Version).major
#Build Object & Assign Values
$MyObject = [PSCustomObject]@{
'Server Name' = ($Env:Computername)
'Operating System' = ($OS)
'PowerShell Version'= ($PSVersion[0])
'IPv4 Address' = ($IPAddress)
}
$MyObject | Format-List
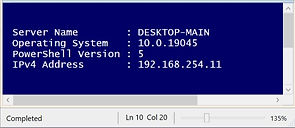